I am trying to automate the year selection slider on the CroplandCROS website (https://croplandcros.scinet.usda.gov/) using Run JavaScript in Automation Anywhere (AA).
Approach Tried:
I wrote the following JavaScript code to move the slider dynamically by calculating the correct position based on the target year:
(function() { var slider = document.querySelector("div[role='slider']"); var track = document.querySelector(".esri-slider__track"); if (slider && track) { var targetYear = 2015, minYear = 1997, maxYear = 2023; var trackRect = track.getBoundingClientRect(); var posX = ((targetYear - minYear) / (maxYear - minYear)) * trackRect.width; var targetX = trackRect.left + posX; var sliderRect = slider.getBoundingClientRect(); var startX = sliderRect.left + sliderRect.width / 2; function moveSlider(stepX) { var eventMove = new PointerEvent("pointermove", { bubbles: true, cancelable: true, composed: true, clientX: stepX, clientY: trackRect.top + trackRect.height / 2 }); slider.dispatchEvent(eventMove); } var pointerDown = new PointerEvent("pointerdown", { bubbles: true, cancelable: true, composed: true, clientX: startX, clientY: trackRect.top + trackRect.height / 2 }); slider.dispatchEvent(pointerDown); let currentX = startX, stepSize = (targetX - startX) / 20; function animateMove() { if (Math.abs(currentX - targetX) < Math.abs(stepSize)) { moveSlider(targetX); setTimeout(() => { var pointerUp = new PointerEvent("pointerup", { bubbles: true, cancelable: true, composed: true, clientX: targetX, clientY: trackRect.top + trackRect.height / 2 }); slider.dispatchEvent(pointerUp); }, 100); } else { currentX += stepSize; moveSlider(currentX); setTimeout(animateMove, 10); } } setTimeout(animateMove, 50); } else { console.error("Slider or track element not found."); } })();
Observations:
-
If I open the website in a New Tab, select Last used browser tab, and choose Google Chrome, the script works fine, and the slider moves correctly.
-
However, when I open the browser using New Window, select Google Chrome, and pass the website link, the script does not execute and gives the following error in Run JavaScript:
Error:
Browser: Run JavaScript Executes JavaScript function in a web page or in an iFrame within a web page (Supported browsers only) To run JavaScript in iFrame, use Recorder package 2.5.0 or above (Chrome and Edge only) Required bot agent version: 21.210 or above
Troubleshooting Attempts:
- Assigned the CroplandCROS website to a window variable (
$Window3$
) and passed it to Run JavaScript, but the error still persists. - Ensured the bot agent version and Recorder package are up to date.
Expected Outcome:
- When opening the browser using New Window and passing the website link, it should allow Run JavaScript to execute properly within the same window.
Help Needed:
- How can I make sure Run JavaScript executes properly in a new browser window in AA?
- Are there any AA-specific configurations required to allow JavaScript execution in a newly opened window?
- Are there better approaches to automate this slider, perhaps using a different method within AA?
Any guidance or alternative solutions would be greatly appreciated! 🚀
Ps: I am attaching the screenshots of both working and not working approach.
This is the Screenshot of the slider i want to autmate:
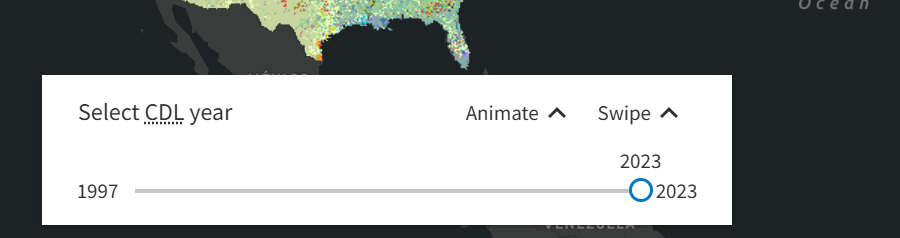