Getting “bot Error” for Code.
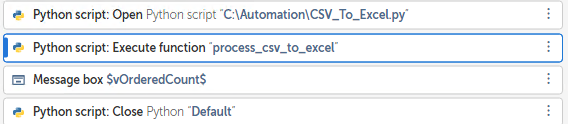
Steps:
- Convert to .py File.
- Open using A360 Commad
- Use Execute Function and call the function “process_csv_to_excel”
- Closed Session
Code:
import pandas as pd
import openpyxl
import os
def process_csv_to_excel():
csv_file_path = "C:/Automation/Test.csv"
excel_file_path = "C:/Automation/Test.xlsx"
try:
# Read the CSV file
read_file = pd.read_csv(csv_file_path, on_bad_lines='skip', encoding='utf-8')
# Write to Excel
read_file.to_excel(excel_file_path, index=None, header=True)
success_message = "Conversion successful."
except Exception as e:
success_message = f"An error occurred: {str(e)}"